Getting Started with Redis: A Beginner's Guide
Beginner guide to Redis, covering installation, basic commands, and fundamental concepts to help you get started.
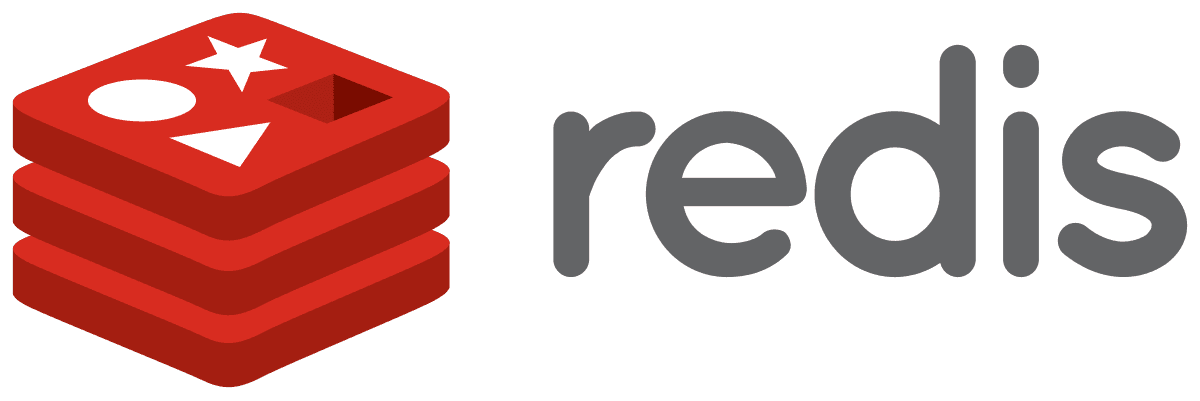
Introduction
What is Redis? Redis is an open-source, in-memory database that's used for caching, message brokering, and more. Why is Redis popular? Redis is popular because it is extremely fast as it stores data in memory rather than on a disk.
Installing Redis
To install Redis on your local machine, you can follow the instructions on the official website. In this blog post, we will install Redis on a WSL (Windows Subsystem for Linux) environment using Ubuntu.
# Update the package list
sudo apt update
# Install Redis
sudo apt install redis-server
# Check the status of Redis
sudo systemctl status redis-server
# Test Redis using redis-cli
redis-cli ping # Expected output: PONG
Database
Redis is a NoSQL database that stores data in key-value pairs. Each key is unique and maps to a value. Redis supports various data types such as strings, lists, sets, hashes, and more. The database concept is same as relational database (mysql, postgres, and etc) but the way of storing data is different.
In Redis we can also create a database and store data in that database. But it kind of different from relational database where we usually create database by name, in redis database is created by number. By default redis has 16 databases and we can switch to any database by using SELECT
command.
# Select database 0 (default selecred database is 0)
SELECT 0
# Select database 1
SELECT 1
# Select database 19
SELECT 19
Basic Redis Commands
Here are some basic Redis commands that you can use to interact with the Redis server.
Connecting to Redis CLI
Redis CLI is a command-line interface that allows you to interact with the Redis server. To connect to the Redis CLI, simply run the following command:
redis-cli
Storing, retrieving and other basic commands
This are some basic commands to store, retrieve, and delete data in Redis.
# Store data
SET mykey "Hello, Redis!"
# Retrieve data
GET mykey # Expected output: "Hello, Redis!"
# Check if a key exists
EXISTS mykey # Expected output: 1 (true)
# Delete a key
DEL mykey
# Append key value
APPEND mykey "This is appended data" # Expected output: 13
# List all keys matching the pattern
keys pattern # ex: keys * (list all keys)
Expiration
By default, keys in Redis do not expire, it will be stored until it is deleted or the server is restarted. Sometimes there a case where we want to store data only for a certain period of time, lets say like we want to make a cache. Redis provides a way to set expiration time for a key so we don't have to worry about deleting it manually. This are some commands to set expiration time for a key.
# Set an expiration time for a existing key (in seconds)
EXPIRE mykey 60 # Key will expire after 60 seconds
# Set an expiration time for a new key
SETEX mykey2 60 "Hello, Redis!" # Create a key with value and expiration time
# Check the time to live (TTL) of a key
TTL mykey # Expected output: 55 (remaining time in seconds)
Increment and Decrement
Redis provides commands to increment and decrement the value of a key. This is useful for implementing counters and other similar functionalities. So we don't have to worry about race condition when multiple clients are trying to increment or decrement the value of a key.
# Increment the value of a key
INCR mycounter # Expected output: 1
# Decrement the value of a key
DECR mycounter # Expected output: 0
# Increment the value of a key by a specific amount
INCRBY mycounter 5 # Expected output: 5
# Decrement the value of a key by a specific amount
DECRBY mycounter 2 # Expected output: 3
Flush or Clear Database
Redis provides a command to flush or clear the entire database. This will remove all keys and their values from the database.
# Flush the entire database
FLUSHALL
# Flush the current database
FLUSHDB
Pipeline
Redis provides a feature called pipeline that allows you to send multiple commands to the server in a single request. This can improve performance by reducing the number of round trips between the client and the server. This is like a batch operation where we can send multiple commands in a single request.
# Pipeline operation using redis-cli
redis-cli -h host -p port -n database --pipe < commands.txt
# Example of commands.txt (bulk insert)
SET key1 value1
GET key1
SET key2 value2
GET key2
Transactions
Like relational databases, Redis also supports transactions. You can group multiple commands into a single transaction. However, unlike relational databases, Redis transactions are not executed immediately, they are added to a queue. Then, you can execute or discard the transaction.
# Start a transaction, BEGIN in SQL
MULTI
# Add commands to the transaction
SET key1 value1 # Ouput: QUEUED
# Execute the transaction, COMMIT in SQL
EXEC
# Discard the transaction, ROLLBACK in SQL
DISCARD
Monitor
Sometimes we want to see what commands are being executed on the server. Redis provides a MONITOR
command to see all the commands being executed on the server.
# Listen for all requests received by the server in real-time
MONITOR
Server Information
You can get information about the Redis server using the INFO
command. This will provide details such as memory usage, connected clients, and more.
# Get information about the Redis server
INFO
# Get information about memory usage
INFO parameter_name # ex: INFO memory
# Get value of configuration parameter from redis.conf
CONFIG GET parameter_name # ex: CONFIG GET port
Client Connection
Redis save all client information in server, so we can get information about all connected clients. This is useful to debug/see how many clients are connected to the server and what they are doing.
CLIENT LIST # Get information about all connected clients
CLIENT ID # Get your client ID, used in Client
CLIENT KILL ip:port # Kill a client connection
Protected Mode
Redis by default listen to all network interfaces, so it can be accessed from any IP address. To secure the server, Redis has a protected mode that is enabled by default. In protected mode, Redis only allows connections from localhost.
To setup which IP address can connect to the server, we can set bind
parameter in redis.conf
file. We also need to make sure the protected-mode
parameter is set to yes
, so the server only allows connections from IP addresses specified in the bind
parameter.
BIND 127.0.0.1 -::1 # Only allow connections from localhost
protected-mode yes # Only allow connections from IP addresses specified in the bind parameter
Security
Redis provides various security features to protect the server from unauthorized access and attacks. Some of the key security features include authentication and authorization.
Authentication
Redis also provides a way to secure the server using a password. This is useful to prevent unauthorized access to the server. Redis authentication procress is fast because it run in memory, so we need a long/complex password so it will be hard to be brute forced.
Authorization
Authorization is a procress to give access for user who has been authenticated. Redis provide a way to restrict access to certain commands using the ACL
(Access Control List) feature, here is the rules documentation. ACL is useful to prevent unauthorized users from executing sensitive commands.
Security Implementation
# create a default user with no password and permissions, so we can login to the server
user default on +@connection
# Create a user with password and permissions
user [username] on [command] [key] [pattern] [password]
user admin on +@all ~* >adminpassword # Ex: Admin user with all permissions
# Choose username and password (Login)
AUTH [username] [password]
Working with Persistence
Redis provides different persistence options to save data to disk. The two main options are RDB snapshots and AOF (Append Only File).
RDB Snapshots
RDB snapshots are point-in-time snapshots of your dataset. You can configure Redis to take snapshots at specified intervals. This the official documentation.
save 900 1 # Save the dataset to disk if at least 1 key changed in 900 seconds
# The saved file will be named dump.rdb and stored in the same directory as the Redis configuration file by default
# You can change the directory and filename using the following configuration
dir ./ # Save the file in the current directory
dbfilename dump.rdb # Save the file with a custom name
We also can trigger a snapshot manually using the SAVE
command or BGSAVE
command. The SAVE
command will block the server until the snapshot is complete, while the BGSAVE
command will run in the background.
SAVE # Save the dataset to disk (blocking/synchronous)
BGSAVE # Save the dataset to disk (non-blocking/asynchronous)
AOF (Append Only File)
Snapshotting is not very durable. If your computer running Redis stops, your power line fails, or you accidentally kill -9 your instance, the latest data written to Redis will be lost. So append-only file is an alternative, fully-durable strategy for Redis. AOF is a log of every write operation received by the server, and the log is replayed during server startup, reconstructing the original dataset. This is the official AOF documentation.
Eviction Policies
Redis is an in-memory database, so it has a limited amount of memory. When the memory is full, Redis can evict (delete) keys to make space for new data. Redis provides various eviction policies to determine which keys to evict. By default, Redis uses the noeviction
policy, which means it will return an error when the memory is full. So we need to configure the eviction policy in the redis.conf
file. This is the official evection policies documentation.
maxmemory 10mb # Set the maximum memory limit
maxmemory-policy allkeys-lfu # Least Frequently Used (LFU)
> CONFIG SET maxmemory 100mb # set maxmemory at runtime using redis-cli
Redis in a Programming Language
You can interact with Redis using various programming languages. Here is an example using Python:
import redis
# Connect to Redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Set a key
r.set('foo', 'bar')
# Get a key
value = r.get('foo')
print(value) # Expected output: b'bar'
Advanced Features (Optional)
Redis also offers advanced features such as Pub/Sub messaging, Lua scripting, and Redis Streams. These features can be explored as you become more comfortable with Redis.
Conclusion
Redis is a powerful and versatile in-memory database that can be used for various purposes such as caching, message brokering, and more. By understanding the basics and exploring its advanced features, you can leverage Redis to build high-performance applications.
Related Articles
Memahami Docker dari Dasar
Pengenalan Docker dari konsep dasar hingga penggunaan Docker yang efektif
Introduction to Network Programming
An introduction to Network programming, covering basic concepts and practical usage